Working with strings#
String indexing and slicing#
We have already looked briefly at strings previously. Like lists, strings too are of Sequence type in Python, therefore they support slicing, membership operators, concatenation, replication and the other utility functions we mentioned in the Lists section. Instead of data items, however, strings are composed of a sequence of characters. Indexes for str “Hello World!” below shows an example of the sequence of characters in the string “Hello World!” together with its index positions.

Fig. 7 Indexes for str “Hello World!”#
Thus, we can extract characters from a string as in the code below:
s = "Hello World!"
print("s at index 6 is", s[6])
#Accessing s with a negative index
print("s[-2] value is", s[-2])
s at index 6 is W
s[-2] value is d
And we can slice a string as below:
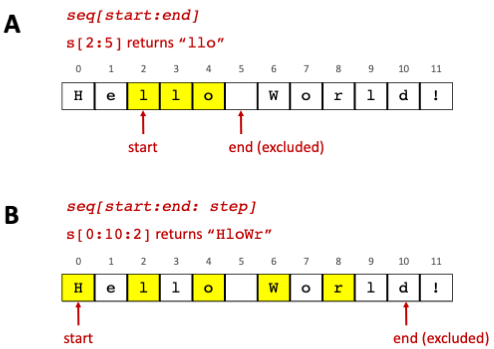
Fig. 8 String slicing.#
Strings are immutable#
Strings are immutable, one cannot change the contents of a character in a string. Trying to modify a character in a string will throw an error as shown below:
s[1] = 'a'
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[2], line 1
----> 1 s[1] = 'a'
TypeError: 'str' object does not support item assignment
String utility functions and methods#
More useful functions and methods for strings are listed in String utility functions. A list of all the String methods in Python can be found here.
Function |
Description |
---|---|
|
returns the length of the string |
|
returns |
|
returns |
|
returns |
|
returns the number of times |
Exercise 4 (Joining strings)
Level:
Given a list of str
objects defined as below:
words = ["keep", "calm", "and", "carry", "on"]
Join all the str
objects together and separate them by a space into one str
object and print it.
The final string should have the following value: “keep calm and carry on”
Tip
Use the join()
String method. Look at its documentation
to see how this can be used.
Solution to ( 4
The join()
method takes as an argument a list of strings to join together. The separator to use between the different data items in
words
is the string we are calling the join()
method on.
words = ["keep", "calm", "and", "carry", "on"]
quote = " ".join(words)
print(quote)
keep calm and carry on
Exercise 5 (Word counting - splitting strings)
Level:
In this exercise write code that counts and prints the number of words in the famous quote from Star Wars: “May the Force be with you”
Solution to ( 5
To divide strings by a separator, the split()
string method provides an easy way to do this. Have a look at the
documentation. If no arguments are passed
to the split()
method, all whitespace is removed from the string that called split()
and a list is returned with the
separated strings.
sentence = "Your path you must decide"
words = sentence.split()
print(f"There are {len(words)} words in the words list.")
print(words)
There are 5 words in the words list.
['Your', 'path', 'you', 'must', 'decide']